Unity Test Framework Tutorial (Unity 2022.3)
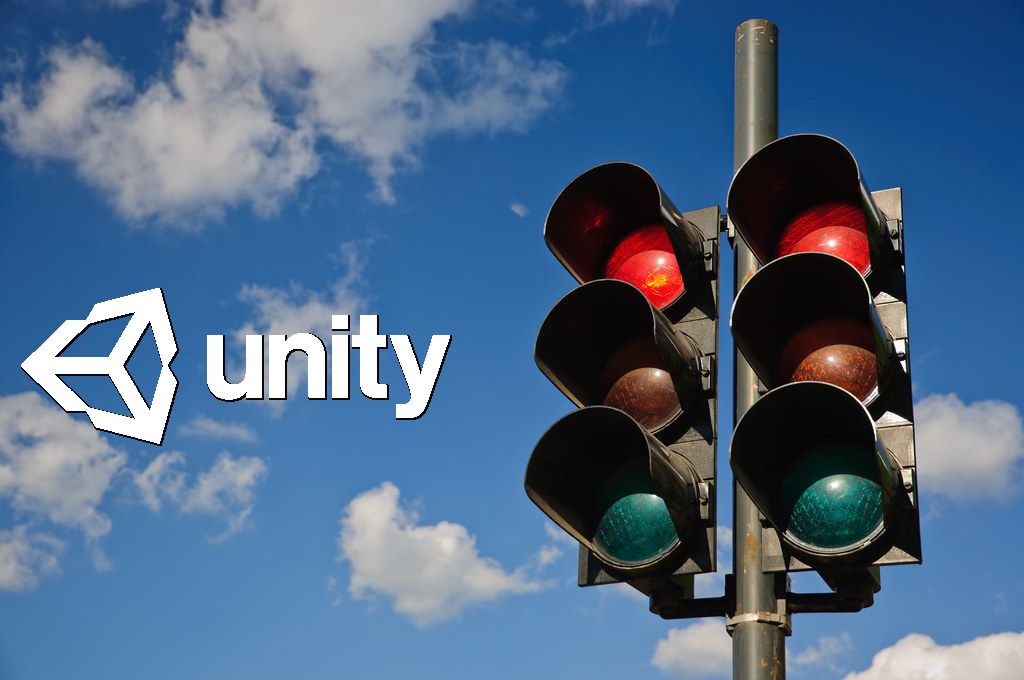
This tutorial has been updated in September 2024 for Unity 2022.3.
Testing is an important part of game development. Manual testing is often the obvious way. But manual testing can be very repetitive. That's why automated game testing is a very powerful tool for any development team.
If you’re asking yourself “how do you write automated tests with unity?”, this tutorial is for you. At the end of it you will know:
- How to set up the Unity Test Framework
- How to write your first test with it
- How to simulate inputs with the Unity InputSystem
Table of Contents
The Sample Project
For this tutorial, we will use a sample project available on Github. Download the project by clicking Code > Download Zip.
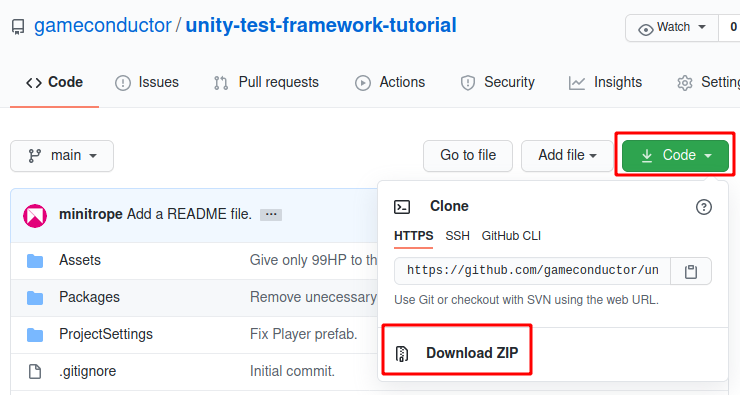
Open the project with Unity (it might take some time). Once the editor is ready, open the Scenes/Main.unity
scene.
Launch the scene and play the game to have a sense of the gameplay mechanics. The game is a simple action game in which skeletons are chasing you. You can kill them by using your sword (left click), your telekinesis power (right-click), or your shockwave power (hold the middle button then release).
You can reset the game by pressing the r
key.
Installing the Unity Test Framework
The Unity Test Framework (UTF) is a package maintained by Unity. It is available via the package manager.
To Install the package, go to Window > Package Manager. Search Test Framework via the search bar, and install the release version (1.1.33 when writing this tutorial).
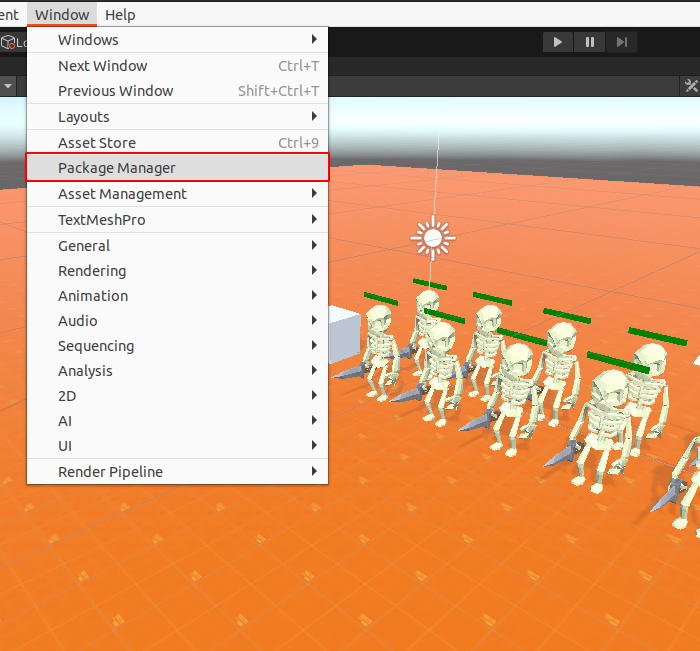
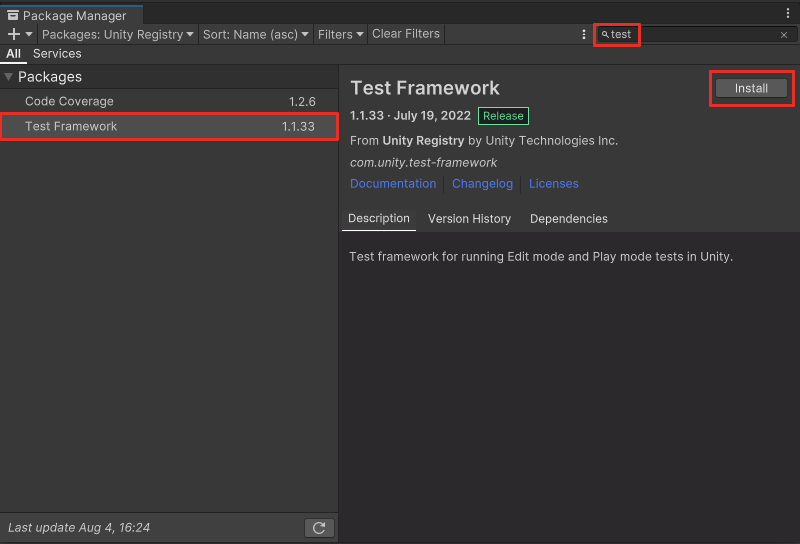
Setting up the Test Assembly
Installing the framework gives you access to a new panel under Window > General > Test Runner:
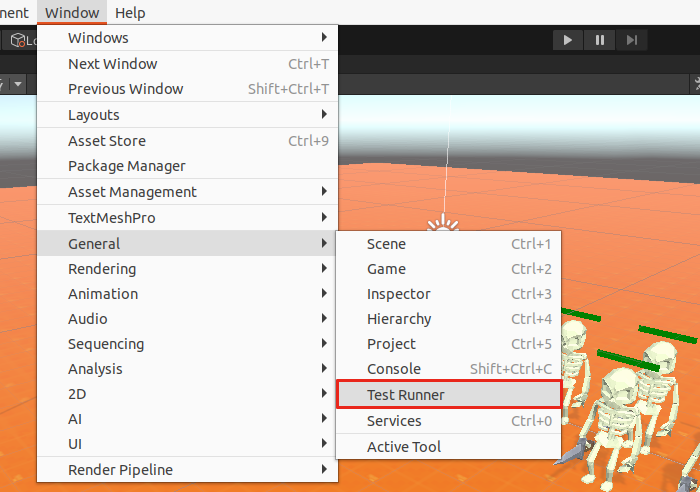
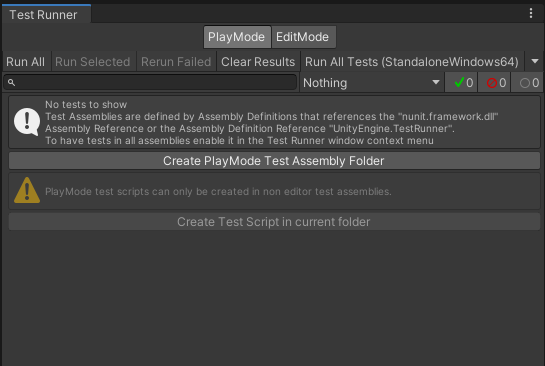
The runner has 2 main tabs PlayMode and EditMode. The PlayMode is for tests that will run while the game is playing. EditMode tests run in the Unity Editor and do not need the game to be playing. These tests have access to the Editor code besides the game code. It is thus more suitable for Editor extensions.
You want to write tests that are as close as possible to the final game build. So using the PlayMode will likely be what you want.
To start writing PlayMode tests, go to your Assets Folder and then click Create PlayMode Test Assembly Folder in the runner. This should create a new Folder, named Tests by default.
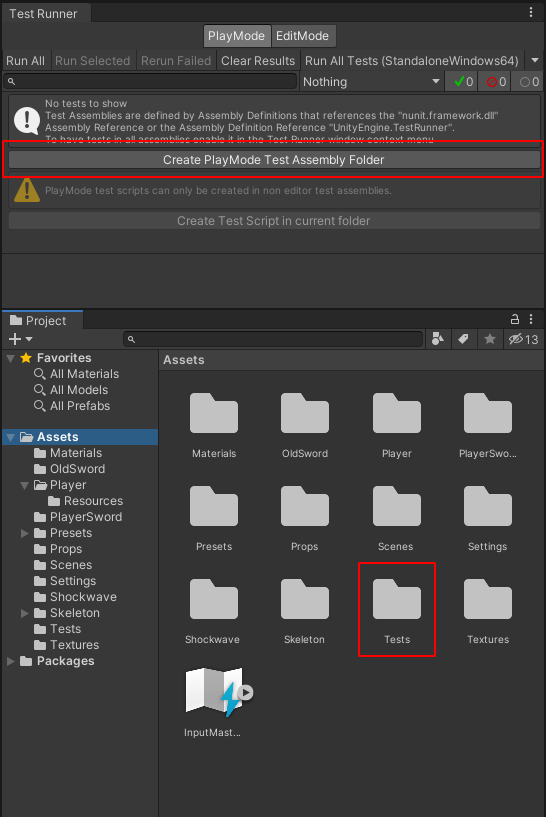
Inside the folder, you will find Tests.asmdef
which is the assembly definition for your tests. Your tests will need to access the game code. To achieve this, we create another assembly for the rest of the code and then link it in Tests.asmdef
.
Go to your Assets folder, and with the contextual menu Create > Assembly Definition. Give a name to the file (for example GameAssembly
).
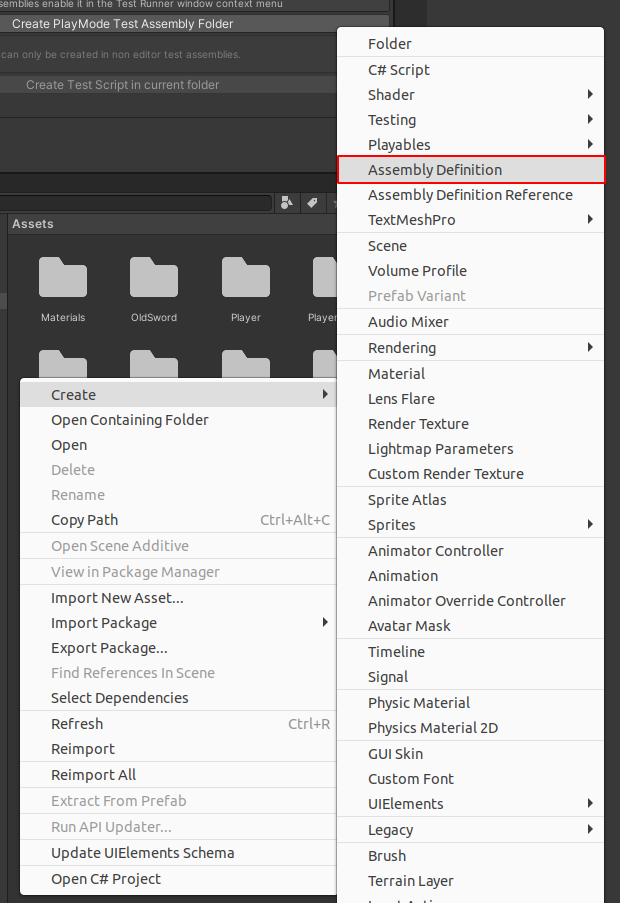
This will associate the entire game code with the new assembly. The code depends on the InputSystem
so you need to reference it in the new assembly. Open GameAssembly.asmdef
in the inspector, and add Unity.InputSystem
in the references. Apply the changes:
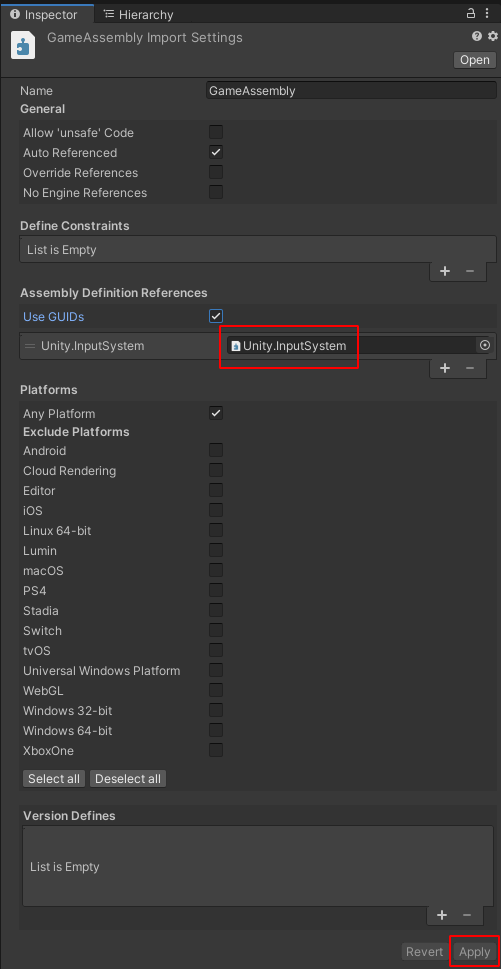
Now come back to your Tests
assembly. In the inspector add GameAssembly
to the Assembly Definition References. Don’t forget to save the modification.
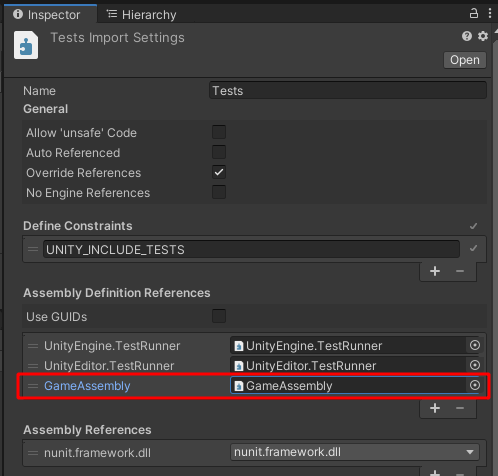
A note on the different kind of tests
Before starting to write any tests for your game you need to ask yourself what kind of tests you want. UTF is just a framework, and only provides the tools to write tests with code. The kind of tests you will write is up to you.
If you’re not familiar with testing, you might start with Unit Testing. But there are many more types of tests. Integration tests, end-to-end tests, or performance tests are a few more examples.
If you want to learn more about this you can refer to the following links:
- The different types of software testing (Atlassian)
- Software Testing Types (Software Testing Fundamentals)
In the rest of the tutorial we will first write a very basic unit test. Then we will move forward with more useful integrations tests.
Your First test
Finally, we can start writing the first test. To do so, we will create a test script via the Test Runner.
Go to your Tests folder and select the Tests
assembly definition. In the runner, click Create Test Script in the current folder. Name it TestSuite
and open the file. The content should look like this:
We will test if a player has the right amount of HP and if using the method applyDamage
changes it.
Add a member to the class to reference the player prefab:
Rename the first test to TestPlayerDamage
, and instantiate a player:
The test will now appear in the Test Runner. Even if the test itself is not useful yet, you can already run it. In the runner, select the test in the tree and click Run Selected.
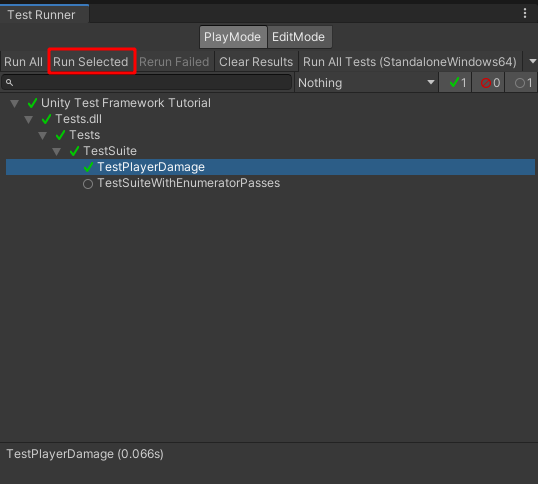
Since the test doesn’t check anything, it passes. Add an assertion to check if the player has 100 HP:
Run this test and see it fail. It's because the default HP for the player prefab is 99, not 100.
Select Prefabs/Resources/Player.prefab
and change its Health to 100 via the inspector. Run the test again and this time it turns green.
Now we want to make sure using the applyDamage
method on the player changes its HP. To do so, call the method on the player and add another assertion. The complete test now looks like this:
Run the test again and this time it passes with the new assertion. This means the applyDamage
method changes the player HP as expected.
Congratulations! You wrote your very first Unity test.
A better test: attacking the skeleton
The previous test is not very useful, because it tests a very tiny part of the game. For something more useful, we will check if attacking the skeleton affects its HP.
This test has the following requirements:
- Having access to the player and skeleton prefabs
- Run without blocking the game loop
- Being able to simulate player input
To fulfill them, first we need to add the skeleton prefab to the TestSuite
class:
UnityTest
attribute, which lets us use coroutines:Now let’s instantiate a player and a skeleton:
Note that yielding a value is necessary because UnityTest
requires an enumerator. And since we're not using any wait yet, we simply yield a null value.
Additionally, the skeleton depends on a player object, so we give it one:
To allow our test to use and simulate inputs, we need to inherit from the InputTestFixture
class:
However this class is not available by default. So we need to add the relevant references to our tests assembly.
Go to your tests folder and select the Tests
assembly definition.
Under Assembly Definition References, add Unity.InputSystem
and Unity.InputSystem.TestFramework
. Apply the modifications:
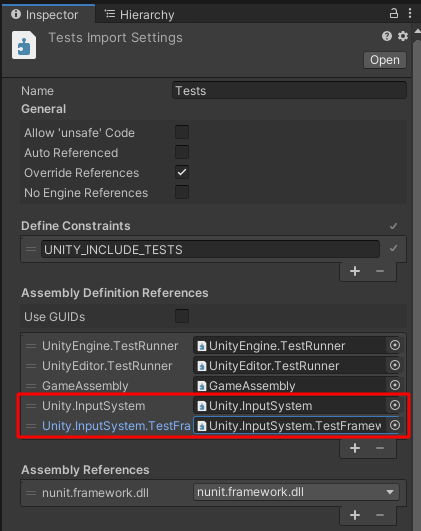
After this, references to InputTestFixture
shouldn’t raise compilation errors. But we need further modifications to simulate input. We have to add a mouse and a keyboard device to our InputSystem
. We do that by overriding the Setup
method of our class:
Now we can write the important part of the test! We will check the initial skeleton’s HP, trigger a sword slash, and check if the HP have changed. The entire test then looks like this:
Using Scenes for integration testing
Since the default testing scene has no floor, the player and the skeleton are free falling. This situation can lead to test flakiness, that's why we stopped gravity in the previous test. A better test would provide a proper environment so it's closer to an actual gameplay configuration.
To improve that we will use a test scene, providing a default environment. This scene is already available in the project as Scenes/Sandbox.unity
, we just have to load it in our Setup method:
Note that the scene has to be available in the build settings. Or else you’ll see the following error:
Scene 'Scenes/Sandbox' couldn't be loaded because it has not been added to the build settings or the AssetBundle has not been loaded. To add a scene to the build settings use the menu File->Build Settings...
You can create similar scenes in advance to test the behavior of your objects, and then load them from the tests.
Optionally and to make things slightly better, we will use the scene’s camera instead of the player’s camera. This makes it easier to see what’s happening. To do that, we tell the Player we won’t use its camera, in the TestSlashDamagesSkeleton
test method:
We will also disable the skeleton since we’re only testing damage and not its behavior:
Finally, here is the entire TestSuite.cs
file:
Conclusion
Getting started with the Unity Test Framework is not a simple task. However I hope you learned how to write your first meaningful tests with this tutorial.
If you're interested in automated game testing, don't hesitate to browse through the blog, as more resources are added regularly.
External links: